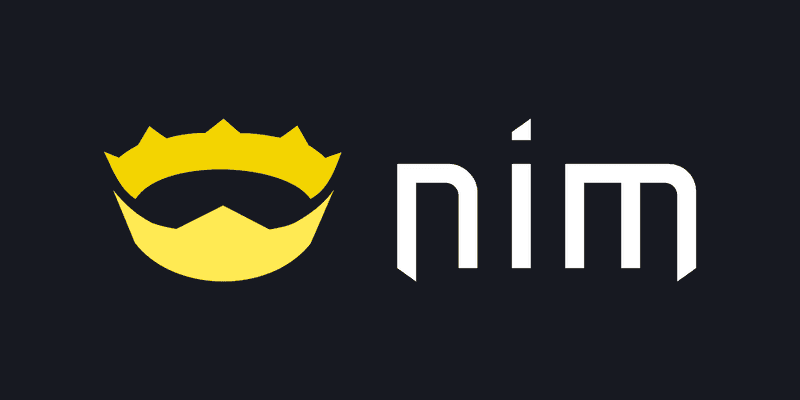
Nim: Data Structures
Mar 21, 2022
More complex data structures can be defined via a type
statement:
typeState = enumVictoria, NewSouthWales, Queensland, Tasmania, SouthAustralia, NorthernTerritory, WesternAustralia, ACTvar home = Victoriaecho home# Victoriaecho typeof home# State
Arrays in Nim are of fixed length with their size specificed at compile time. Sequences are like arrays, but have a dynamic length. They can be constructed by the array constructor []
in conjunction with the array to sequence operator @
.
varx: seq[int] # a reference to a sequence of integersx = @[1, 2, 3, 4, 5, 6]
There's also openarrays, though they can only be used as parameters:
proc openArraySize(oa: openArray[string]): int =oa.len
Creating a custom object is done by defining a new type, with a type of object
:
typePerson = objectname: stringage: intvar person1 = Person(name: "Peter", age: 30)echo person1.name # "Peter"echo person1.age # 30
Mark fields that should be visible from outside the defining module with *
.
typePerson* = object # the type is visible from other modulesname*: string # the field of this type is visible from other modulesage*: int
A similar but alternate approach for object-like behaviour is to use tuples:
typePerson = tuple[name: string, age: int]varperson: Personperson = (name: "Peter", age: 30)
Hi, I'm Glenn! 👋 I've spent most of my career working with or at startups. I'm currently the Director of Product @ Ockam where I'm helping developers build applications and systems that are secure-by-design. It's time we started securely connecting apps, not networks.
Previously I led the Terraform product team @ HashiCorp, where we launched Terraform Cloud and set the stage for a successful IPO. Prior to that I was part of the Startup Team @ AWS, and earlier still an early employee @ Heroku. I've also invested in a couple of dozen early stage startups.
Previously I led the Terraform product team @ HashiCorp, where we launched Terraform Cloud and set the stage for a successful IPO. Prior to that I was part of the Startup Team @ AWS, and earlier still an early employee @ Heroku. I've also invested in a couple of dozen early stage startups.