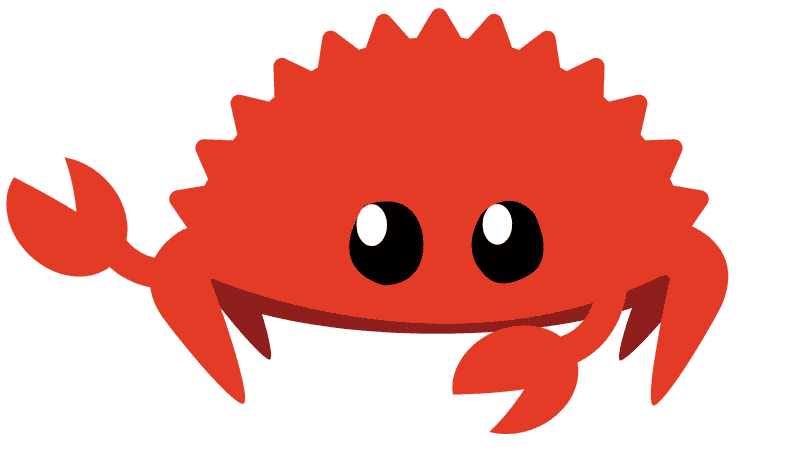
Rust: Concatenating strings
Mar 23, 2022
Another seemingly simple task, another trip down the Rust rabbit-hole to actually understand what's happening. It turns out there's two similar but different types of string: String
and str
. A gross oversimplification that I'm sure will make rustaceans cringe, but is sufficient for my hours-long understanding of how to use Rust: str
is an immutable type, which you access via a reference (e.g. &str
). A String
is for when you know you'll want to own and mutate the string.
With that, we've already seen one way to concatenate string values in our previous exploration of reading and outputting environment variables. The println!
syntax is using the format!
macro's syntax:
fn main() {let a = "Hello";let b = "World!";let c = format!("{} {}", a, b);println!("{}", c);}
Next up is to take advantage of that mutable String
, by declaring a mutable variale and then pushing a new value onto the end of it:
fn main() {let mut a = "Hello".to_string();let b = " World!";a.push_str(&b);println!("{}", a);}
We could also use the concat()
method on an array:
fn main() {let a = "Hello";let b = "World!";let c = [a, " ", b].concat();println!("{}", c);}
Or join()
:
fn main() {let a = "Hello";let b = "World!";let c = [a, b].join(" ");println!("{}", c);}
Previously I led the Terraform product team @ HashiCorp, where we launched Terraform Cloud and set the stage for a successful IPO. Prior to that I was part of the Startup Team @ AWS, and earlier still an early employee @ Heroku. I've also invested in a couple of dozen early stage startups.