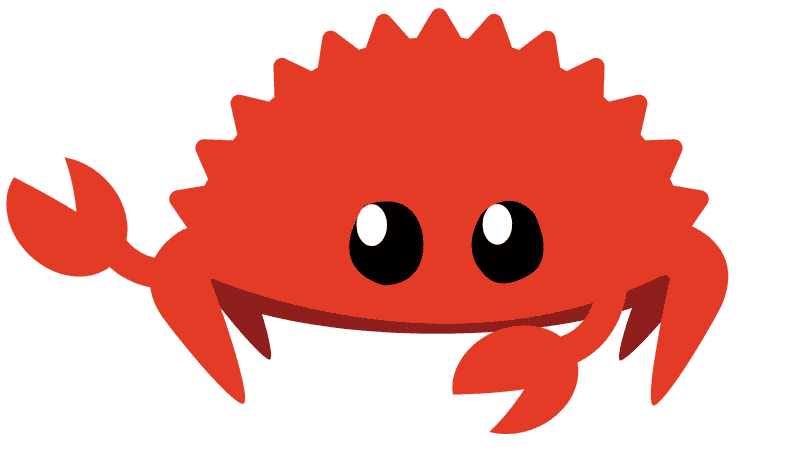
Rust: Data structures
Mar 23, 2022
Rust has a real focus on memory-efficiency as well as memory and thread safety. Which means you can't quite play as fast and loose with variables as you might in a language like Ruby. We've already seen a glimpse of that with the way we had to work with strings. We're going to encounter more of it now with the various data structures we play with.
fn main() {let arr = [1, 2, 3, 4];println!("{}", arr[2]); // 3, because arrays are zero-indexed}
That array is immutable though. We can't add new items. We can't change anything within it. We could do the latter by adding the mut
keyword to the definition but that's only going to be valuable in limited circumstances.
Enter Vectors! Arrays, but growable.
fn main() {let mut arr = vec![1, 2, 3];arr.push(4);println!("arr is now {} items", arr.len()); // Prints 4}
Next up, tuples:
fn main() {let tuple = ("Glenn", 40);println!("{:?}", tuple);println!("{}", name);println!("{}", age);}
Just pairs (or more) of values that you can assign to a variable. You can even have tuples of tuples. Accessing the values is a little trickier as they need to be destructured into it's constituent parts. You'll also see some experimentation with :?
formatter to print the debug output.
Now to some more complicated setups:
#[derive(Debug)]struct Person {name: String,age: u8,}fn main() {let name = String::from("Glenn");let age = 40;let glenn = Person { name, age };println!("{:?}", glenn);println!("{}", glenn.name);println!("{}", glenn.age);}
A struct! It's actually quite familiar to many other languages. Note the introduction of the #[]
compiler hint to tell it how to handle an attempt to print debug info of this struct. Instantiation expects values in the same order if you use the field init shorthand. Or you can be more explicit by providing field: value
definition pairs.
Previously I led the Terraform product team @ HashiCorp, where we launched Terraform Cloud and set the stage for a successful IPO. Prior to that I was part of the Startup Team @ AWS, and earlier still an early employee @ Heroku. I've also invested in a couple of dozen early stage startups.