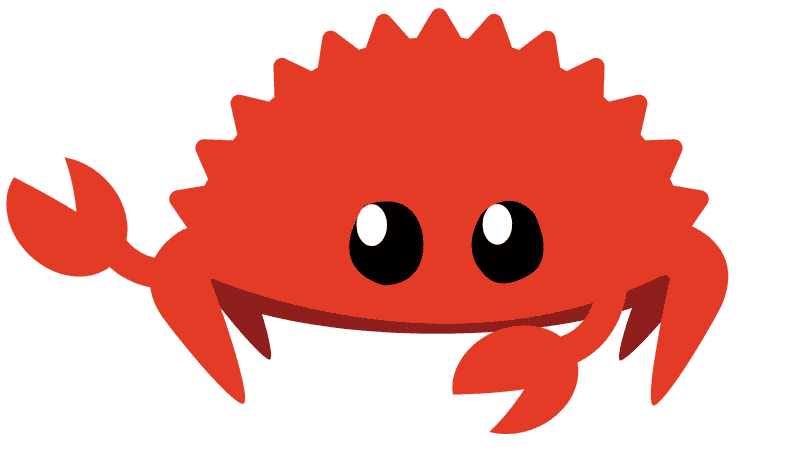
Rust: Making a HTTP POST request (with parameters)
Mar 24, 2022
This one proved to be a little easier given we'd established all of the foundations with a get request:
use hyper::{Body, Method, Client, Request};use hyper::body::HttpBody as _;use tokio::io::{stdout, AsyncWriteExt as _};use url::form_urlencoded;#[tokio::main]async fn main() -> Result<(), Box<dyn std::error::Error + Send + Sync>> {let params = vec![("foo", "bar")];let body = form_urlencoded::serialize(params.into_iter());let client = Client::new();let req = Request::builder().method(Method::POST).uri("http://httpbin.org/post").body(body)?;let mut resp = client.request(req).await?;println!("Response: {}", resp.status());while let Some(chunk) = resp.body_mut().data().await {stdout().write_all(&chunk?).await?;}Ok(())}
We've had to unravel the previous get()
request into two parts now: 1) building out the request objects and then 2) passing the request into the client to execute it. Adding on to that Request::builder
is how and where we'd add additional headers or otherwise manipulate the request before sending it.
Hi, I'm Glenn! 👋 I've spent most of my career working with or at startups. I'm currently the Director of Product @ Ockam where I'm helping developers build applications and systems that are secure-by-design. It's time we started securely connecting apps, not networks.
Previously I led the Terraform product team @ HashiCorp, where we launched Terraform Cloud and set the stage for a successful IPO. Prior to that I was part of the Startup Team @ AWS, and earlier still an early employee @ Heroku. I've also invested in a couple of dozen early stage startups.
Previously I led the Terraform product team @ HashiCorp, where we launched Terraform Cloud and set the stage for a successful IPO. Prior to that I was part of the Startup Team @ AWS, and earlier still an early employee @ Heroku. I've also invested in a couple of dozen early stage startups.