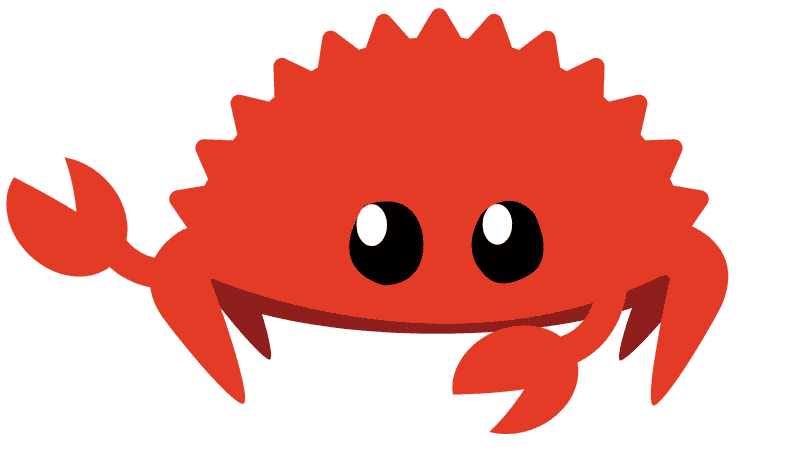
Rust: Extracting substrings
Mar 23, 2022
As in Nim, Rust will let you access strings via positional location/slice:
fn main() {let a = "Hello World!";let b = &a[0..];println!("{}", b); // Hello World!let c = &a[0..1];println!("{}", c); // Hlet d = &a[..5];println!("{}", d); // Hellolet e = &a[6..];println!("{}", e); // World!}
Though it's worth mentioning this is a slice of bytes, so unicode characters would take two. It's also not difficult to make this approach panic, so there's more than enough reasons to suggest it's probably not what you want to do most of the time.
A similar approach, but with the ability to better handle the error cases is to call get()
instead of accessing the slice directly. In this example, for the sake of readability and showing like-for-like, I'm going to call unwrap()
on each example. Better would be to take the previous lessons on error handling and apply them here:
fn main() {let a = "Hello World!";let b = a.get(0..).unwrap();println!("{}", b); // Hello World!let c = a.get(0..1).unwrap();println!("{}", c); // Hlet d = a.get(..5).unwrap();println!("{}", d); // Hellolet e = a.get(6..).unwrap();println!("{}", e); // World!}
For finding a substring within a string, there are the find()
and rfind()
methods. The latter giving the result starting from the right (end) of the string:
fn main() {let a = "Hello World!";let b = a.find("World").unwrap();println!("{}", b); // 6}
If you're expecting to find multiple matches there is matches()
and match_indices()
which will return an iterator for going over results:
fn main() {let a = "Hello World! Hello Rustaceans! Hello Everyone!";for (idx, matched) in a.match_indices("Hello") {println!("Found {} at index {}", matched, idx);}}
Would output:
Found Hello at index 0Found Hello at index 13Found Hello at index 31
You could then use the index from this method to do whatever byte position based substring access you wanted.
Previously I led the Terraform product team @ HashiCorp, where we launched Terraform Cloud and set the stage for a successful IPO. Prior to that I was part of the Startup Team @ AWS, and earlier still an early employee @ Heroku. I've also invested in a couple of dozen early stage startups.