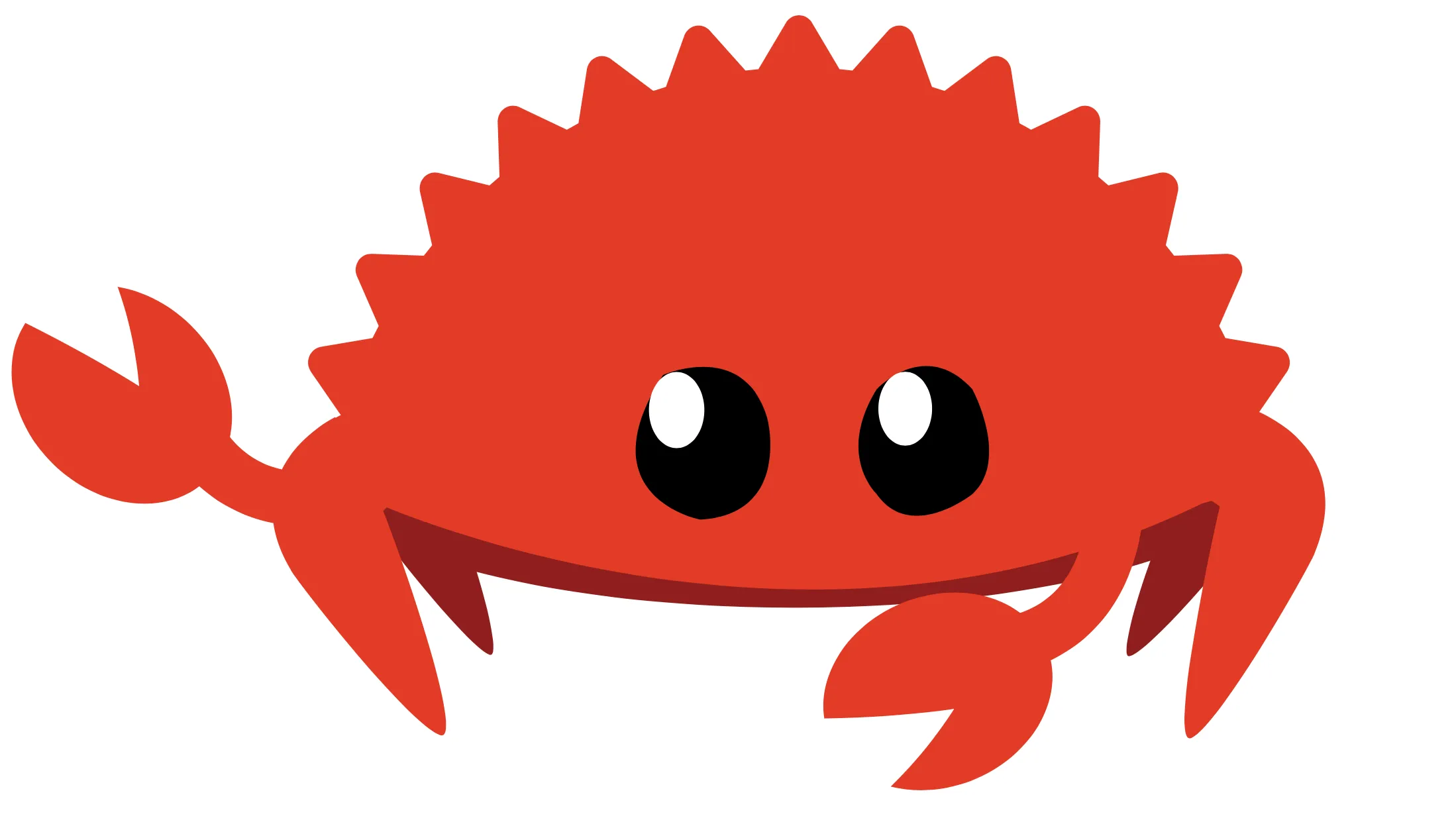
Rust: Create a basic web/HTTP server
For the web server we’re going to use the same HTTP library we used for making requests, Hyper
. The Hyper docs actually have a really great guide for creating a server which is exactly what this has been based off. Here’s my version:
use hyper::{Body, Request, Response, Server, Method, StatusCode};
use hyper::service::{make_service_fn, service_fn};
async fn echo(req: Request<Body>) -> Result<Response<Body>, hyper::Error> {
match (req.method(), req.uri().path()) {
// Serve some instructions at /
(&Method::GET, "/") => Ok(Response::new(Body::from(
"Welcome! Try a GET to /hello",
))),
(&Method::GET, "/hello") => Ok(Response::new(Body::from(
"World!",
))),
// Simply echo the body back to the client.
(&Method::POST, "/echo") => Ok(Response::new(req.into_body())),
// Return the 404 Not Found for other routes.
_ => {
let mut not_found = Response::default();
*not_found.status_mut() = StatusCode::NOT_FOUND;
Ok(not_found)
}
}
}
async fn shutdown_signal() {
// Wait for the CTRL+C signal
tokio::signal::ctrl_c()
.await
.expect("failed to install CTRL+C signal handler");
}
#[tokio::main]
async fn main() -> Result<(), Box<dyn std::error::Error + Send + Sync>> {
let addr = ([127, 0, 0, 1], 3000).into();
let service = make_service_fn(|_| async { Ok::<_, hyper::Error>(service_fn(echo)) });
let server = Server::bind(&addr).serve(service);
println!("Listening on http://{}", addr);
let graceful = server.with_graceful_shutdown(shutdown_signal());
// Run this server for... forever!
if let Err(e) = graceful.await {
eprintln!("server error: {}", e);
}
Ok(())
}
Published: 25/03/2022
Hi, I'm Glenn! 👋
I've spent most of my career working with or at startups. I'm currently the VP of Product / GTM @ Ockam where I'm helping developers build applications and systems that are secure-by-design. It's time we started securely connecting apps, not networks.
Previously I led the Terraform product team @ HashiCorp, where we launched Terraform 1.0, Terraform Cloud, and a whole host of amazing capabilities that set the stage for a successful IPO. Prior to that I was part of the Startup Team @ AWS, and earlier still an early employee @ Heroku. I've also invested in a couple of dozen early stage startups.
I've spent most of my career working with or at startups. I'm currently the VP of Product / GTM @ Ockam where I'm helping developers build applications and systems that are secure-by-design. It's time we started securely connecting apps, not networks.
Previously I led the Terraform product team @ HashiCorp, where we launched Terraform 1.0, Terraform Cloud, and a whole host of amazing capabilities that set the stage for a successful IPO. Prior to that I was part of the Startup Team @ AWS, and earlier still an early employee @ Heroku. I've also invested in a couple of dozen early stage startups.