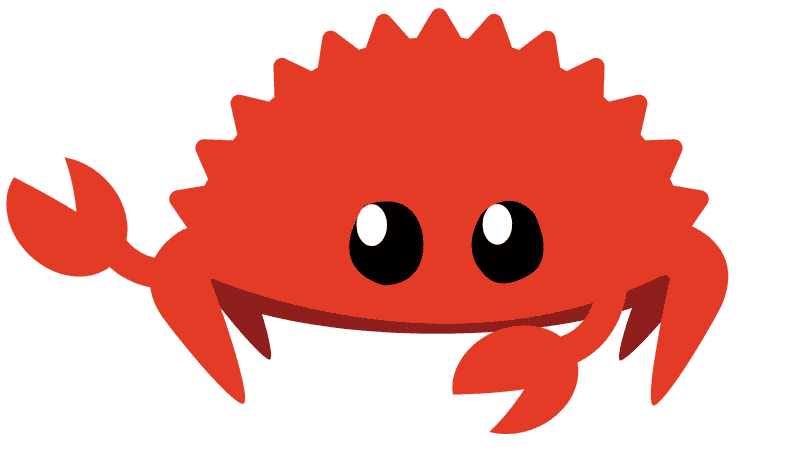
Rust: Parsing JSON
Mar 24, 2022
Enter the world of serializer/deserializers, or serdes. While you can build your own, it's probably no great suprise that Rust has one for JSON available out of the box:
use serde_json;fn main() {let data = r#"{"first_name": "Glenn","last_name": "Gillen","age": 40}"#;let json: serde_json::Value = serde_json::from_str(data).expect("JSON was not well-formatted");println!("{}", json)}
We have to provide an explict type annotation here on the assignment to the json
variable. The type of serde_json::Value
is a convenient way to convert untyped JSON values by saying it can basically be any of the 6 different possibilities a JSON value could represent.
To parse into a structured object/type means first defining they struct. Then we use the #[derive]
macro we saw earlier for enabling debug output, which we'll use again here, but this time we'll add the Serialize
and Deserialize
helpers. Finally we change the type annotation from the generic serde_json::Value
to our desired type of Person
:
use serde::{Deserialize, Serialize};use serde_json;#[derive(Serialize, Deserialize, Debug)]struct Person {first_name: String,last_name: String,age: u8,}fn main() {let data = r#"{"first_name": "Glenn","last_name": "Gillen","age": 40}"#;let p: Person = serde_json::from_str(data).expect("JSON was not well-formatted");println!("{:?}", p)}
Previously I led the Terraform product team @ HashiCorp, where we launched Terraform Cloud and set the stage for a successful IPO. Prior to that I was part of the Startup Team @ AWS, and earlier still an early employee @ Heroku. I've also invested in a couple of dozen early stage startups.